Project 2 - CUDA, CUDA, CUDA
Due 10/20 at
11:59pm
Chicago time
This
project will focus on using CUDA for an n-body simulation of the
collision of our Milky Way galaxy and the Andromeda galaxy about 5
billion years from now. Galaxy collisions are nice since you have a lot
of particles affected by gravity and the odds are that none of those
individual particles will actually collide during the simulation.
There
is a nice paper here on the topic of modelling galaxy interactions as
background:
http://www.kof.zcu.cz/st/dis/schwarzmeier/galaxy_interactions.html
There
is a nice dataset available here containing 82,000 particles for a
Milky Way /Andromeda collision:
http://bima.astro.umd.edu/nemo/archive/#dubinski
To
make the collision more obvious we can use just the disk and bulge
locations and avoid the halo. That still leaves 49152 stars. OpenGL can
easily draw that many stars but the gravity calculations will need to
be simplified to do them in realtime.
Chapter
31 of GPU-gems 3 "Fast N-Body Simulation with CUDA" deals with this
topic in detail so that would be a good place to
look before you start coding this in CUDA. This chapter is provided as
part of the n-body CUDA demo.
CUDA
will be dealing with computing and updating the particle positions.
OpenGL will be used to display the results. Initially you should see
something like this:

as the simulation progresses the two galaxies will pass through each
other and their structures will rapidly break down with lots of stars
flying all over the place, but then gravity will pull the remnants back
together into a new strutcure.
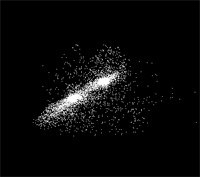
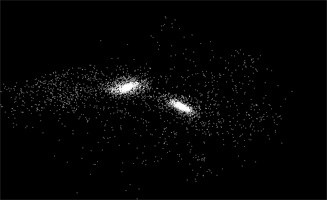
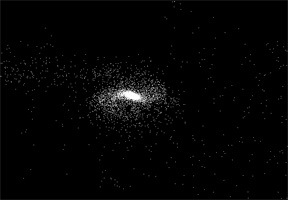
Your first job
is to write CUDA and OpenGL code to make the galaxy collision happen
realistically within a reasonable amount of time. As part of this you
should allow the user to dynamically change their viewpoint of the
interaction through rotation and zoom. You should not need pan but you
can add it if you want. You should allow the user to choose various
colour schemes
(e.g. each galaxy has a different colour, or the different parts of the
galaxy have differnet colours.) One colour scheme I found useful in
degugging was using r, g, and b to show the velocities of individual
stars in the x, y, and z directions, since that makes it easier to see
what direction groups stars are moving in and to see when their
velocities begin to change during the collision.
The capability of your graphics card will determine how many stars you
can compute with. I would suggest starting off by reading in and just
displaying all the stars to make sure you have the data read in
correctly, then pick a subset of the stars to work with (e.g. every
10th star, every 5th star, etc) in CUDA. You may also want to stagger
the computations so not every star is updated every iteration. The
particle demos from class should be a good starting point.
Your next job is
to work on optimizing this code and investigate how various parameters
affect the efficiency of CUDA. A major focus here is documenting your
efforts so you can explain
to the class how you improved the speed of your code (or increased the
number of particles you could compute with.)
You should then
create some more scenarios. e.g. instead of a full collision maybe its
a near miss, or just the edges collide, or the angle of the collision
changes. You could also create more datasets, e.g. a globular
cluster, or a bunch of stars in a plane or the homer simpson
galaxy.
Note that you should not expect to see a nice spiral arm structure in
your visualization. You should see the rotating disc and a compact
central bulge of each galaxy before the collision. You will probably
need to play with some of the gravity parameters to keep the galaxy
structure intact.
As
with the previous project, to turn in your
project you should set up a web page describing your work, including
the well-commented source code and required files to be able to compile
and run your program, and a movie in a common web-viewable format
showing what your
application should look like when its running. You should then email
andy with the location of this website before the deadline. It
would probably be a good idea to put a backup copy of the web page at a
second website just in case I can't get to the first one.
When you send
andy the location of your webpage you should again email a screen
snapshot of your head that is 320 pixels wide by 240 pixels tall in
jpeg format named <your_last_name>.p2.jpg. This image will be
used on the class web pages along with the link to your project web
page.
As with the
previous project, each student
will also give a presentation about their project in-class and
answer some questions about your work. This time the presentation will
be 20 minutes long. You should prepare a good
presentation - you can use your project website, or create some slides.
You should definitely run your code and talk about how you structured
the computation in CUDA. Be sure to practice your presentation so you
finish within the alloted
time so everyone has equal time to present.
last
revision 9/28/08 - added a note that the GPU Gems 3 chapter is
included in the CUDA demo code