(STOLEN FROM TURTLE GEOMETRY)
BEHAVIOR (in 2D)
Basic Movements
- MOVE FORWARD (or BACKWARD) - Relative to the direction it
is facing.
- TURN LEFT (or RIGHT)
Turtle Geometry vs. Coordinate Geometry
- Turtle Geometry properties are Intrinsic
Dependant only upon the figure in question, not on its relation to
its surroundings
- Coordinate Geometry properties are Extrinsic
Based on its relation/orientation to its surroundings
Expressing the Turtle in terms of the Coordinate system
Need to keep some global variables:
- Xpos - the total movement in the X direction
- Zpos - the total movement in the Z direction
- Yrot - the total rotation around the Y axis
This makes turning easy:
- Simply ADD (for LEFT) or SUBTRACT (for RIGHT) the angle
A to Yrot
In order to move forward 1 unit:
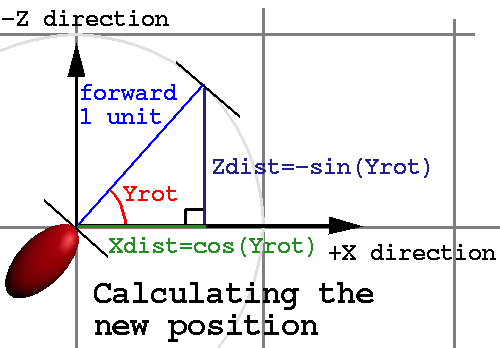
So:
- Xpos = Xpos + (Xdist * SPEED * dt)
- Zpos = Zpos + (Zdist * SPEED * dt)
Where:
- SPEED = how fast you want it to move (1.0 for 1 foot)
- dt = the time since the previous frame - (to keep the motion smooth)
float t;
static float dt, prevtime = 0;
t = CAVEGetTime();
if (t>prevtime && (t-prevtime)<1) dt = t - prevtime;
prevtime = t;
Modeling Smell
Find by Smell
In order to simulate SMELL the Turtle needs to receive information from
the environment. We can then simulate the movement of Turtle to find
food based on its ability to smell the food.
SMELL is based on DISTANCE -- greater DISTANCE = weaker SMELL
Could base movement on the amount of SMELL by using it to calculate the
angle and/or distance to move the Turtle.
Simplest Way
Not based on level of smell, but only on whether the smell is getting
stronger or weaker.
- Continue to move FORWARD
- If SMELL is WEAKER this frame than it was last frame (i.e. the
Turtle is getting farther away from the food) TURN to the RIGHT some
fixed amount
DISTANCE(X1, Z1, X2, Z2)
DX = X2 - X1
DZ = Z2 - Z1
RETURN SQRT(DX^2 + DZ^2)
TO SMELL
IF DISTANCE.TO.FOOD > DISTANCE.LAST.TIME
THEN RESULT = "WEAKER"
ELSE RESULT = "STRONGER"
DISTANCE.LAST.TIME = DISTANCE.TO.FOOD
RETURN RESULT
TO FIND.BY.SMELL2 (TURN)
REPEAT FOREVER
FORWARD 1
IF SMELL = "WEAKER" THEN RIGHT (TURN)
Slightly more interesting way (still very simple)
To make it a bit more realistic, add some random motion
- Continue to move FORWARD
- TURN to the LEFT some random angle in th range
(-RAND.TURN, RAND.TURN)
- Then if SMELL is WEAKER this frame than it was last frame (i.e. the
Turtle is getting farther away from the food) TURN to the RIGHT some
fixed amount
TO FIND.BY.SMELL3 (D1, D2, SMELL.TURN, RAND.TURN)
REPEAT FOREVER
FORWARD RAND (D1, D2)
LEFT RAND (-RAND.TURN, RAND.TURN)
IF SMELL = "WEAKER" THEN RIGHT SMELL.TURN
If we observe the behavior, if the RIGHT TURN Angle remains
constant, as the range of random LEFT TURN Angle increases, the
path degenerates.
Modeling Sight
Receiving information from the environment
In order to simulate vision, the Turtle must again get some information from
the environment. A simple model that is similar to the SMELL examples
above concerns itself with only the intensity of light that reaches the
Turtle's eye. The major difference between these SIGHT and SMELL models
is that SIGHT is directional; it is
dependent upon the Turtle's direction with respect to the stimulus.
Assuming that the Turtle can sense a light source, it needs the ability to
TURN to FACE it.
- DX is the difference in the X coordinates
- DZ is the difference in the Z coordinates
- ARCTAN(DZ/DX) is the total angle of rotation towards the light
- BEARING returns the angle needed to TURN LEFT from current position
in order to face light
- HEADING is the current rotation (Yrot)
TO TOWARDS [PX PZ]
[DX DZ] = [P2X-Xpos -(P2Z-Zpos)]
IF DX > 0 THEN RETURN ARCTAN (DY/DX)
IF DX < 0 THEN RETURN 180 + ARCTAN (DY/DX)
RETURN 90 * SIGN DZ
TO BEARING POINT
RETURN TOWARDS(POINT)-HEADING
TO FACE POINT
LEFT BEARING(POINT)
Keep a Bearing
One possible model is to have the Turtle move while keeping some point
(for instance, a LIGHT) at a fixed bearing.
- FACE the LIGHT
- TURN to the RIGHT some fixed amount
- Move FORWARD 1
TO KEEP.A.BEARING POINT ANGLE
REPEAT FOREVER
FACE POINT
LEFT ANGLE
FORWARD 1
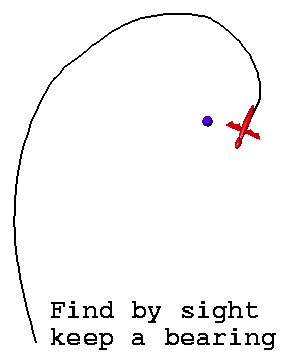
If we observe the behavior, we can see that it causes the Turtle to
spiral around the LIGHT
Two-eye Model
The Turtle could also use vision to face a point. It has two eyes, each
with its own field of vision. Depending on the angles that define these
fields of view for each eye, there may be some overlap where both eyes
can see.
- BEARING again returns the angle needed to TURN LEFT from
current position in order to face point
TO RIGHT.EYE.SEES POINT
IF BEARING(POINT) > 300 THEN RETURN "TRUE"
IF BEARING(POINT) < 10 THEN RETURN "TRUE"
RETURN "FALSE"
TO LEFT.EYE.SEES POINT
IF BEARING(POINT) > 350 THEN RETURN "TRUE"
IF BEARING(POINT) < 60 THEN RETURN "TRUE"
RETURN "FALSE"
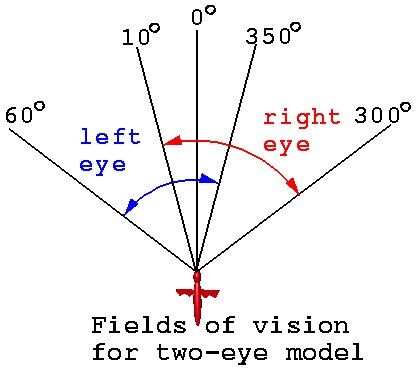
Head for a Point
- If the point lies in the field of vision for either eye, then the Turtle
is headed in roughly the right direction. move FORWARD.
- If the point is not in the field of view, TURN to the
LEFT until the point is once is view.
TO HEAD.FOR POINT
REPEAT FOREVER
IF EITHER
LEFT.EYE.SEES(POINT) ,
RIGHT.EYE.SEES(POINT)
THEN FORWARD 10
ELSE LEFT 10
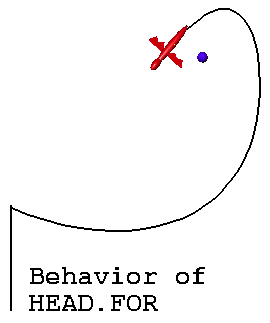
Two-eye Model with Intensity
Increasing the amount of information received from the environment
creates a more sophisticated criterion for making decisions about which
direction to travel in order to reach a desired destination. We will now
modify to include INTENSITY of a light source, not only whether
it falls within the field of vision.
INTENSITY is dependant on:
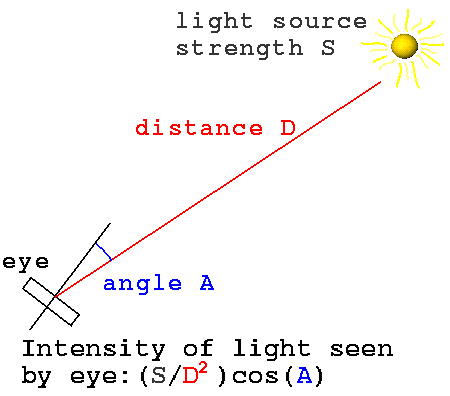
TO INTENSITY.LEFT SOURCE
IF NOT LEFT.EYE.SEES(SOURCE) THEN RETURN 0
FACTOR = STRENGTH / (DIST(SOURCE)^2)
ANGLE = BEARING (SOURCE) - 45
RETURN (FACTOR * COS(ANGLE))
TO INTENSITY.RIGHT SOURCE
IF NOT RIGHT.EYE.SEES(SOURCE) THEN RETURN 0
FACTOR = STRENGTH / (DIST(SOURCE)^2)
ANGLE = BEARING (SOURCE) + 45
RETURN (FACTOR * COS(ANGLE))
*note that the ANGLE computation includes an offset of 45 degrees
(negative for the LEFT eye and positive for the RIGHT eye) from the
Turtle's heading.
To include INTENSITY in a SIGHT model simply:
- Calculate the INTENSITY for the LEFT eye
- Calculate the INTENSITY for the RIGHT eye
- Continue to move FORWARD trying to keep the INTENSITY
balanced between the eyes
- If the INTENSITY of the LEFT eye is greater, TURN to the LEFT some fixed amount
- If the INTENSITY of the RIGHT eye is greater, TURN to the RIGHT some fixed amount
TO FIND.BY.SIGHT
REPEAT FOREVER
FORWARDS 1
IF INTENSITY.LEFT(SOURCE) > INTENSITY.RIGHT(SOURCE)
THEN LEFT 10
ELSE RIGHT 10
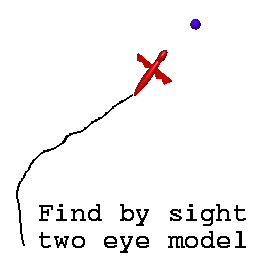
Some Possible Variations
- Give eyes different strengths
- Add random motion to TURN direction
- Add random distance to FORWARD amount (vary the speed randomly)
- Add multiple light sources with varying strengths
- Add multiple Turtles with varying attributes
- Some that seek out others (predators)
- Some that avoid others (prey)
Sources for more information
Turtle Geometry
Abelson, Harold and Andrea A. diSessa
c. 1980 by The Massachusetts Institute of Technology
part of The MIT Press Series in Artificial Intelligence
Look here for more sources soon!
back to NOW
back to
insley on the internet